## Constructing an # ALU --- CS 130 // 2021-11-10 ## Administrivia - Assignment 4 + Due tomorrow night (due to my error) - Assignment 5 is released + Will talk about it at the end of class today # Questions ## ...about anything? # ALU ## The ALU - What does ALU stand for? + Arithmetic Logic Unit - What does an ALU do? + Does all the logical and arithmetic operations of the CPU ## Basic ALU - The simplest ALU conceivable is one that only operates on two 1-bit inputs and can perform only two operations: **AND** and **OR** ```py def basic_alu(a, b, op): if op == 0: return (a and b) else: return (a or b) ``` - Let's implement this in Logisim! ## Including Addition as an Op - For Assignment 4, you were implementing a full adder like the one below: 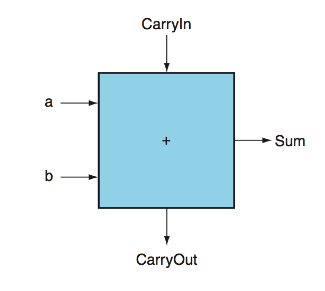 ## Including Addition as an Op - We can change our ALU so that when `op = 2`, we will do addition instead of AND or OR ```py def basic_alu(a, b, c_in, op): if op == 0: return (a and b) elif op == 1: return (a or b) elif op == 2: return (a + b + c_in) # 2-bits, c_out and result ``` - Let's implement this in Logisim! # Multi-Bit ALU ## Including Other Operations - How can we include **subtraction** as an op? - How can we include **NAND** as an op? ## Including Other Operations - This version of the 1-bit ALU has inputs: + `$a$`, `$b$`, `$a_\text{inv}$`, `$b_\text{inv}$`, `$c_\text{in}$`, and `$\text{op}$` - What are each of the inputs if I want to compute: + `$a$` plus `$b$` + `$a$` minus `$b$` + `$a$` AND `$b$` + `$a$` NAND `$b$` + NOT `$a$` # `slt`