# The Datapath --- CS 130 // 2021-11-29 ## The Datapath - What is a "datapath" and what does it consist of? + It is the main component of the CPU that executes instructions + Fetches instructions and data from memory, performs arithmetic, etc. + Consists of ALUs, a register file, RAM, and various supplementary logic gates ## Instruction Execution 1. Uses the PC to fetch the next instruction to be executed from memory 2. Identifies instruction type and registers involved and fetches their contents from the register file 3. Depending on the instruction: - Uses the ALU to do appropriate arithmetic - Read/write RAM for load/store - Update PC to PC + 4 or the jump target address ## K&S Datapath Demo - [K&S Datapath Simulator](javascript:window.open('http://users.dickinson.edu/%7Ebraught/kands/KandS2/datapath.html','KSA','width=525,height=650');) - [K&S Simulator with Main Memory](javascript:window.open('http://users.dickinson.edu/%7Ebraught/kands/KandS2/dpandmem.html','KSB','width=800,height=650');) - [K&S Simulator with Microprogramming](javascript:window.open('http://users.dickinson.edu/%7Ebraught/kands/KandS2/micromachine.html','KSC','width=850,height=650');) - [K&S Computer Simulator](javascript:window.open('http://users.dickinson.edu/%7Ebraught/kands/KandS2/machine.html','KSC','width=850,height=650');) # Building a Datapath ## Instruction Fetch 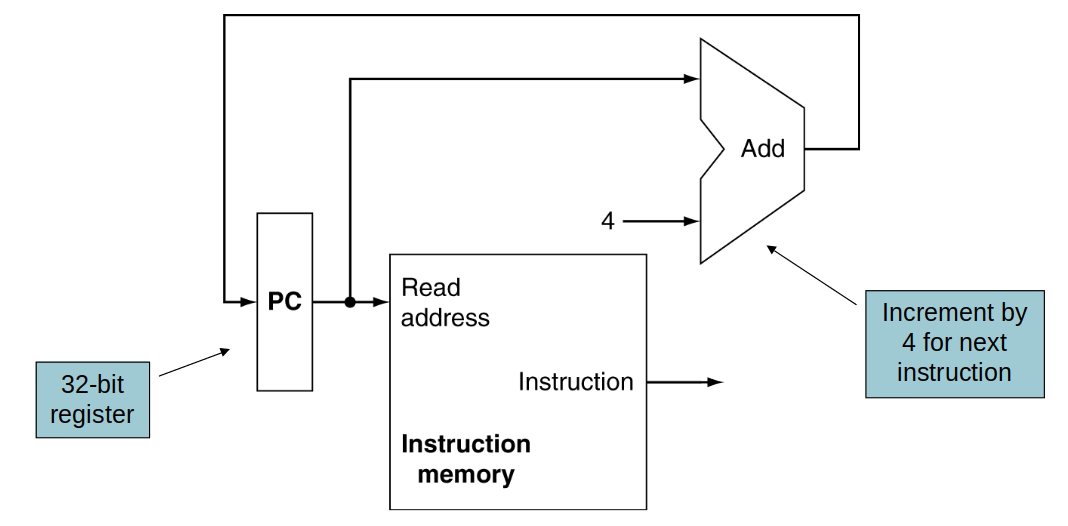 ## R-Format Instructions - Read two register operands, perform ALU operation, write result to target register 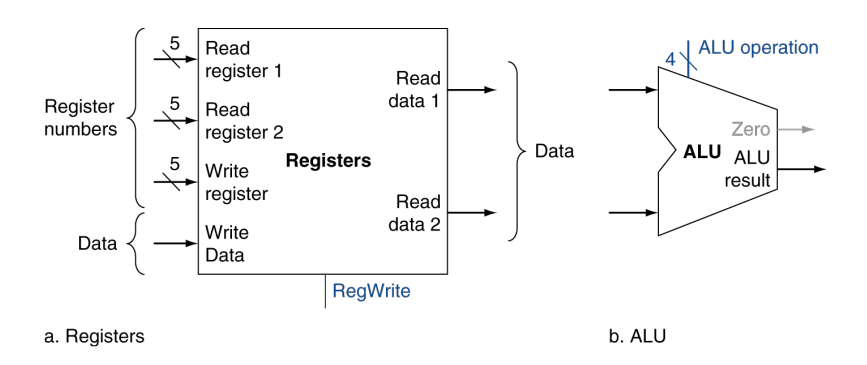 ## Load/Store Instructions - Read register operands, calculate address, read/write to memory 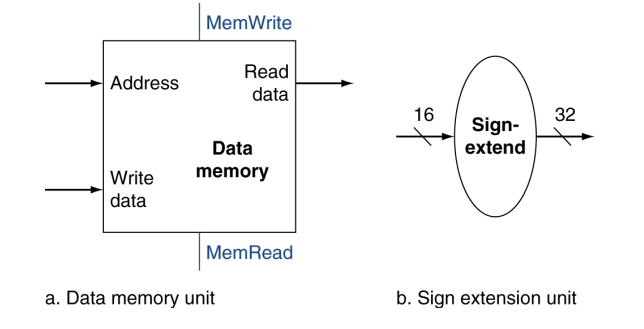 ## Branch Instructions - Read register operands, compare operands, calculate target address 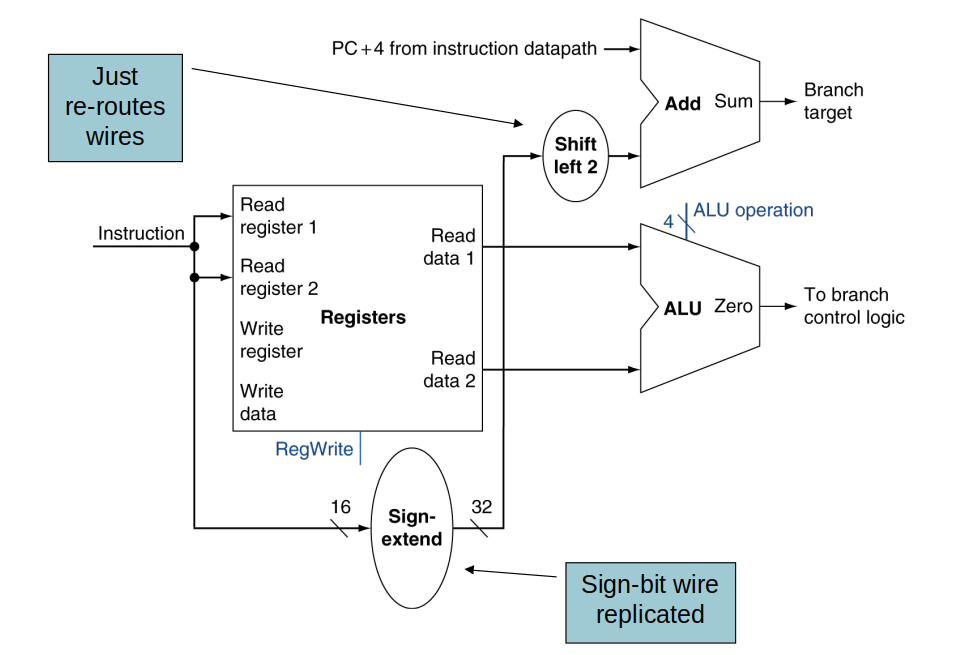 ## The Full Datapath
# Control ## ALU Control - This datapath has an ALU opcode of four bits - We will be using the following operations: ---
ALU Control
Function
0000
AND
0001
OR
0010
add
0110
subtract
0111
set-on-less-than
1100
NOR
## ALU Control - When executing a load word or store word, what ALU function will be used? + It will use `add` to compute the memory address - When executing a branch instruction, what ALU function will be used? + It will use `subtract` to compare the values of the two registers (it has a `Zero` output) - When executing an R-type instruction, the ALU function depends on the 6-bits of the `funct` code ## ALU Control 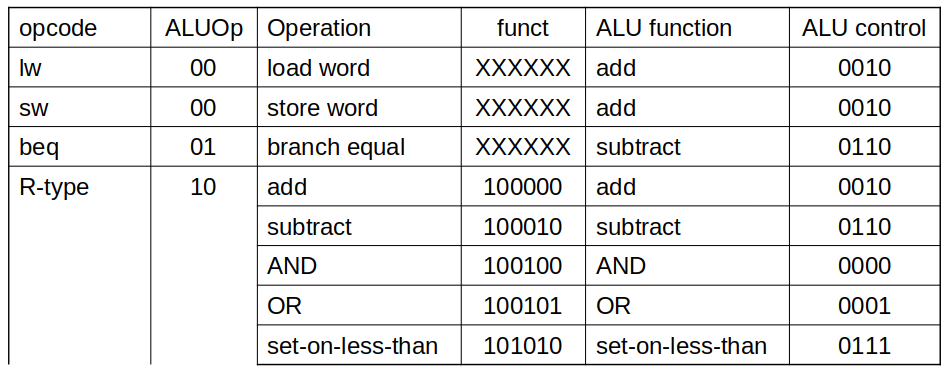 ## ALU Control 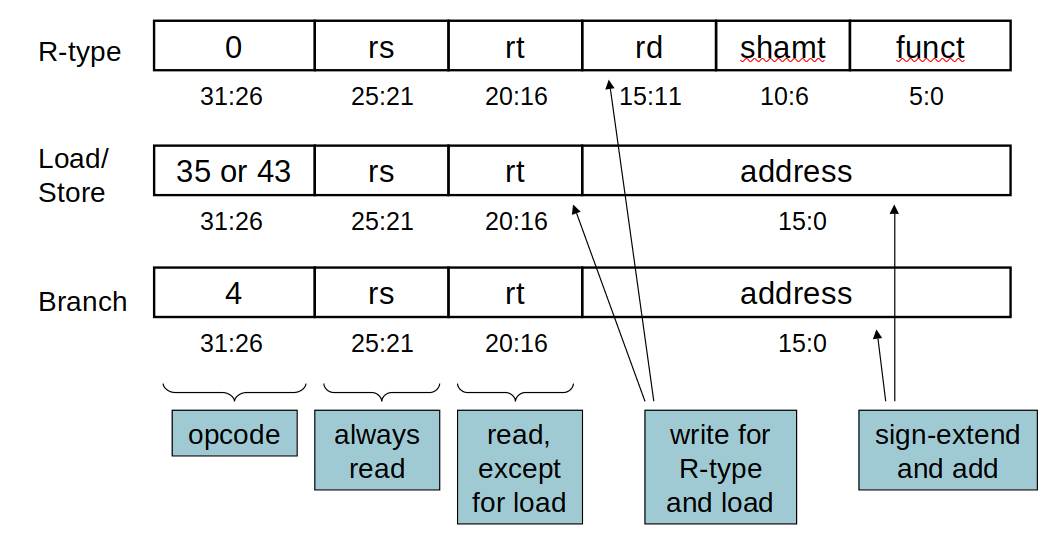 ## Datapath with Control
## Control Truth Table - The control unit can be implemented with a simple combinational logic unit from its truth table: 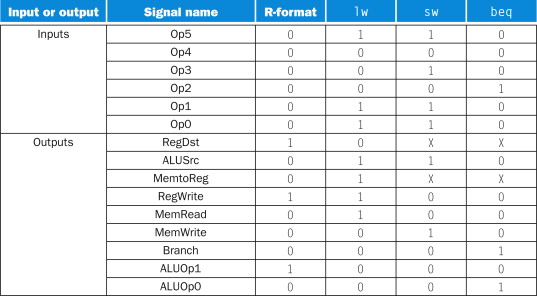 # Performance Issues ## Performance Issues - Longest delay determines clock period + Which instruction takes the most time? + The **load** instruction---5 steps - Not feasible to vary period for different instructions - Violates design principle + Making the common case fast - We will improve performance by **pipelining**