## Introduction to # Graphs --- CS 137 // 2021-10-13 ## Administrivia - Exam 1 debrief # Questions ## ...about anything? # Graphs ## Graph Definition - A **graph** is a linked data structure consisting of: 1. A set $V$ of nodes referred to as **vertices** 2. A set $E$ of **edges** linking vertices together - Sometimes we write $G = (V,E)$ ## Graph Definition
%3
a
a
b
b
a->b
c
c
a->c
b->c
e
e
c->e
d
d
d->c
e->a
--- - $V = \\{a, b, c, d, e\\}$ - $E = \\{(a,b),(b,c),(a,c),(d,c),(c,e),(e,a)\\}$ # Examples ## Dependency Graphs
%3
cs147
CS 147
Graphics
cs167
CS 167
Mach. Learn.
cs178
CS 178
Cloud
cs65
CS 65
CS I
cs65->cs167
cs66
CS 66
CS II
cs65->cs66
cs139
CS 139
Theory
cs65->cs139
cs66->cs147
cs66->cs178
cs146
CS 67
CS III
cs66->cs146
cs130
CS 130
Org I
cs66->cs130
cs135
CS 135
PL
cs146->cs135
cs137
CS 137
Algorithms
cs146->cs137
cs188
CS 188
SE
cs146->cs188
cs191
CS 191
Capstone
math54
MATH 54
Discrete Math
math54->cs167
math54->cs137
math54->cs139
cs83
CS 83
Ethics
cs188->cs191
## Social Networks
%3
Titus
Titus
Tim
Tim
Titus--Tim
Adam
Adam
Titus--Adam
Meredith
Meredith
Meredith--Titus
Meredith--Tim
Meredith--Adam
Eric
Eric
Meredith--Eric
Reza
Reza
Meredith--Reza
Rajin
Rajin
Meredith--Rajin
Adam--Eric
Eric--Tim
Reza--Tim
Chris
Chris
Chris--Titus
Chris--Reza
Chris--Rajin
## City Maps [Des Moines Maps](https://www.catchdesmoines.com/visitor-info/transportation/maps/) # Other Examples? # Flavors of Graphs ## Directed / Undirected - A graph $G = (V,E)$ is an **undirected** graph if the edges are bidirectional + If $(a,b)\in E$, then $(b,a)\in E$ - Otherwise a graph is called **directed** ---
**Directed**
%3
a
a
b
b
a->b
c
c
a->c
b->c
d
d
c->d
d->a
d->c
**Undirected**
%3
a
a
b
b
a--b
c
c
a--c
b--c
d
d
d--a
d--c
## Directed / Undirected - Are city road maps directed or undirected? - What about social networks? ## Weighted / Unweighted - A *weighted graph* has numerical values, called weights, assigned to each edge
dfa
a
a
b
b
a->b
5
c
c
a->c
7
d
d
a->d
13
b->c
3
c->b
11
d->c
17
## Weighted / Unweighted - Can you think of situations where modeling something as a weighted graph could be useful? - Road networks + Speed limits and/or distances as weights - Piping networks + Flow capacities/rates as weights - Power line networks + Edges weighted by the cost to connect two nodes ## Simple / Non-Simple - If a graph supports self-loops and/or duplicate edges it is called **non-simple** - Otherwise they are called **simple**
**Simple**
%3
a
a
b
b
a--b
c
c
a--c
b--c
d
d
d--a
d--c
**Non-Simple**
%3
a
a
a--a
b
b
a--b
c
c
a--c
b--c
c--c
d
d
d--a
d--a
d--c
## Sparse / Dense - If I have a simple graph with $n$ vertices, what is the maximum number of edges possible? + ${n \choose 2} = \frac{n(n-1)}{2}$ - A **dense** graph has many edges, usually $\Omega(n^2)$ - A **sparse** graph has few edges, usually $O(n)$ ## Sparse / Dense  ## Sparse / Dense - Is a road network sparse or dense? + Sparse, because intersections usually have $O(1)$ connections - What about social networks? + Also sparse - Can you think of an example of a **dense** graph? ## Cyclic / Acyclic - An **acyclic** graph does not contain any cycles + Trees are undirected acyclic graphs + Directed acyclic graphs are called **DAG**s
%3
a
a
b
b
a->b
c
c
a->c
b->c
d
d
b->d
c->d
# Social Networks ## The Friendship Graph - Consider a graph where the vertices are people, and there is an edge between two people if and only if they are friends  - What questions can we ask about such a graph? ## Is friendship directed? - Is a friendship graph directed or undirected? + Hopefully undirected! If you are my friend then I hope that I am also your friend! - The "heard of" graph would be directed because many famous people have never heard of me ## Is there a path between us? - Suppose I have a graph of actors/actresses where an edge exists between two people if they've starred in a movie together - What is the length of the shortest path between someone and Kevin Bacon? - A **path** between two vertices is a sequence of vertices that connects one to the other ## Six Degrees of Kevin Bacon 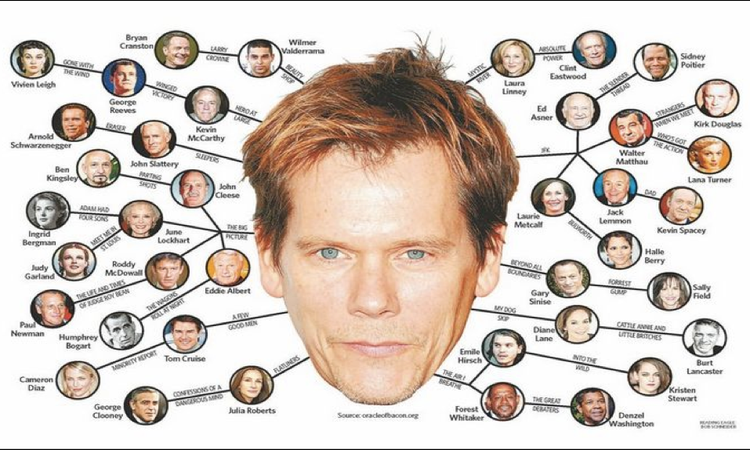 ## Erdős Number - Suppose I have a graph of all published scientists where two scientists share an edge if they have published a paper together - A person's **Erdős number** is the length of the shortest path between them and the Hungarian mathematician Paul Erdős - My Erdős number is 3 ## Who has the most friends? - The **degree** of a vertex is the number of edges connected to it - In directed graphs, each vertex has an **in-degree** and an **out-degree** to keep track of the asymmetry ## What is the largest clique? - A social clique is a group of mutual friends who all hang out together - In a graph, a clique is a fully connected subgraph - Cliques are as dense as possible # Implementing Graphs ## Implementing Graphs - There are two main approaches to implementing a graph $G= (V,E)$ with $n$ vertices ## Adjacency Matrix - We represent $G$ as a $n\times n$ matrix $M$, where $M[i,j]=1$ if $(i,j)$ is an edge and 0 if it isn't - This consumes $\Theta(n^2)$ space - Efficient, but wastes a lot of space for sparse graphs ## Adjacency List - Alternatively we can represent $G$ in a linked way ```java class Vertex
{ V data; List
> neighbors; } ``` - Each vertex stores a list of its adjacent vertices - We can keep track of the vertices in an array - To test if edge $(i,j)$ is in the graph, we need to iterate over $V[i].neighbors$ to see if it contains $j$ ## Adjacency Lists/Matrices Comparison - Which implementation strategy is more efficient for the following operations? --- 1. Testing if $(x,y)$ is in a graph - Adjacency matrix ($O(1)$ instead of $O(n)$) 2. Finding the degree of vertex $x$ - Adjacency list ($O(1)$ instead of $O(n)$) 3. Memory usage on sparse graphs - Adjacency list ($O(n+m)$ instead of $O(n^2)$) ## Adjacency List 4. Memory usage on dense graphs - Adjacency matrix (a small win) 5. Edge insertion or deletion - Adjacency matrix ($O(1)$) 6. Faster to traverse the graph - Adjacency list ($O(n+m)$ instead of $O(n^2)$) --- - An **adjacency list** is best for most applications