# Topological Sort ## and Minimum Spanning Trees --- CS 137 // 2021-10-27 ## Administrivia - Assignment 2 should be turned in + ...but let me know if you need more time ## MathCS Board Game Social - Friday, October 29th, 5:00 to 7:00 PM - Third Floor of Collier-Scripps - Halloween costumes encouraged by not required # Questions ## ...about anything? # DFS and BFS ## DFS and BFS Visualization  ## DFS Implementation ```py def dfs(g, start): discovered = {start} stack = [start] while len(stack) > 0: u = stack.pop() print(u) for v in g[u]: if v not in discovered: discovered.add(v) stack.append(v) ``` ## BFS Implementation ```py[1-12|5,12] from collections import deque def bfs(g, start): discovered = {start} queue = deque(discovered) while len(queue) > 0: u = queue.pop() print(u) for v in g[u]: if v not in discovered: discovered.add(v) queue.appendleft(v) ``` ## Exercise - A directed graph is **strongly connected** if for every pair of vertices, $u,v$, there is a path starting at $u$ and ending at $v$ ## Idea 1: Run DFS a Bunch 1. For each vertex in the graph... - Run DFS starting from that vertex - Check to make sure that every vertex was discovered - If not, return False 2. Return True --- - What is the runtime complexity of this approach? + $O(n\cdot(n+m))$ - Can we do better? ## Idea 2: Run DFS on $G$ and $G_\text{inv}$ - If we run DFS on the original graph $G$, it will tell us if the start vertex can reach every other vertex - If we **invert** the graph $G$ so that all edges are going the opposite direction, running DFS will tell us if the start vertex **can be reached** from every other vertex ## Idea 2: Pseudocode 1. Create a copy of the graph $G$ with all the edges flipped around going the opposite direction 2. Choose a starting vertex $u$ and run DFS on $u$ in the original graph and in the inverted graph 3. If every vertex was discovered both times, return True, otherwise return False # Topological Sort ## Prerequisite Graphs - An issue you might be familiar with is writing a schedule to take all the CS requirements in some particular order (four year plan) ---
%3
cs147
CS 147
Graphics
cs167
CS 167
Mach. Learn.
cs178
CS 178
Cloud
cs65
CS 65
CS I
cs65->cs167
cs66
CS 66
CS II
cs65->cs66
cs139
CS 139
Theory
cs65->cs139
cs66->cs147
cs66->cs178
cs146
CS 67
CS III
cs66->cs146
cs130
CS 130
Org I
cs66->cs130
cs135
CS 135
PL
cs146->cs135
cs137
CS 137
Algorithms
cs146->cs137
cs188
CS 188
SE
cs146->cs188
math54
MATH 54
Discrete Math
math54->cs167
math54->cs137
math54->cs139
cs83
CS 83
Ethics
## Directed Acyclic Graphs - A dependency graph like this is called a **directed acyclic graph** or **DAG** for short - Why doesn't it make sense for a dependency graph to have a cycle? + Cyclical dependencies means some courses cannot be taken without skipping a prerequisite ## Exercise - Write down a sensible ordering for a student to take the following courses ---
%3
cs147
CS 147
Graphics
cs167
CS 167
Mach. Learn.
cs178
CS 178
Cloud
cs65
CS 65
CS I
cs65->cs167
cs66
CS 66
CS II
cs65->cs66
cs139
CS 139
Theory
cs65->cs139
cs66->cs147
cs66->cs178
cs146
CS 67
CS III
cs66->cs146
cs130
CS 130
Org I
cs66->cs130
cs135
CS 135
PL
cs146->cs135
cs137
CS 137
Algorithms
cs146->cs137
cs188
CS 188
SE
cs146->cs188
math54
MATH 54
Discrete Math
math54->cs167
math54->cs137
math54->cs139
cs83
CS 83
Ethics
## Topological Ordering 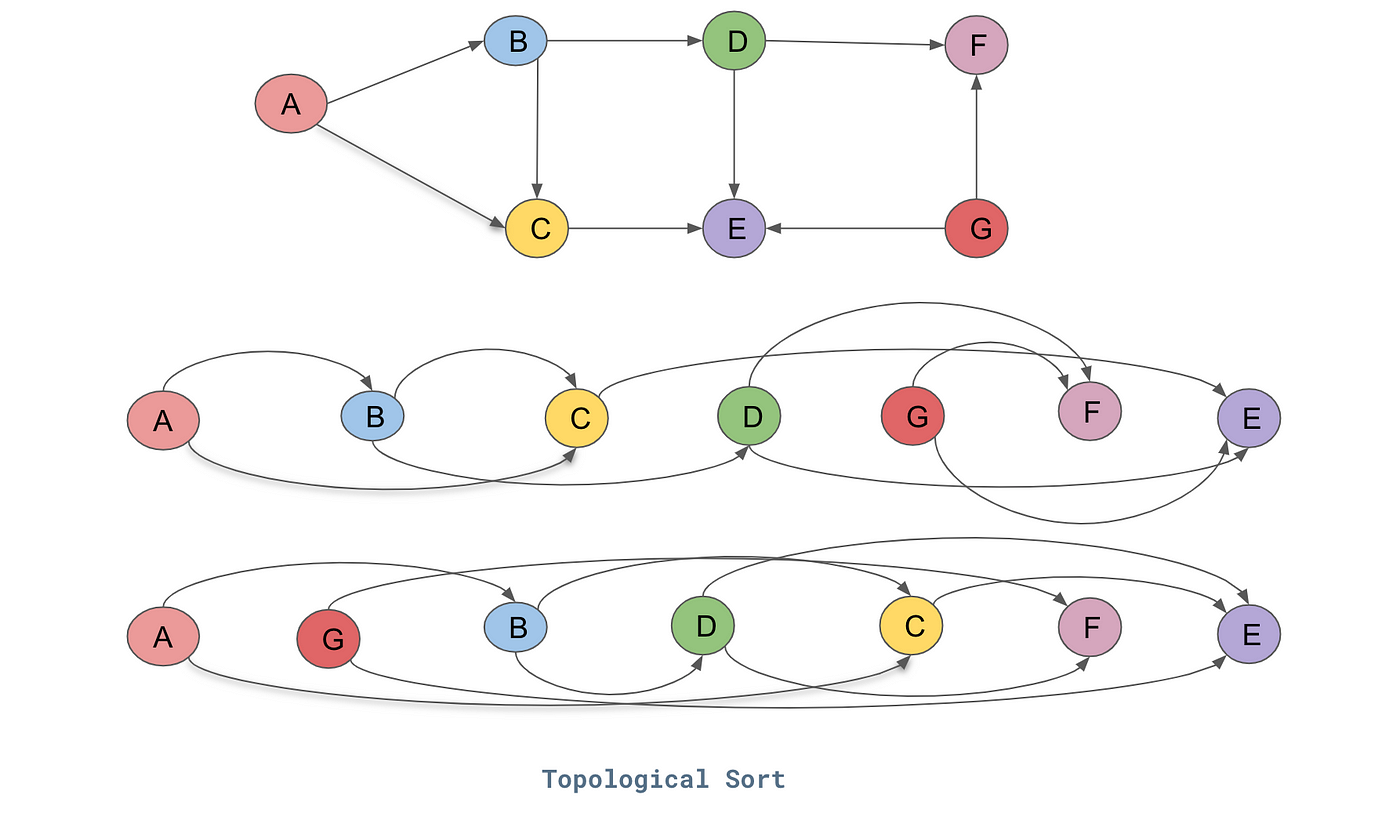 ## Topological Sort - An algorithm for finding a topological ordering is called a **topological sort** - Your process for composing a four-year plan is literally a topological sort - The core idea behind the topological sort algorithm is exactly the process you used to order the courses ## Topological Sort  --- 1. Pick a vertex with in-degree zero (no prerequisites) and add it to our output ordering 2. Remove it from the graph 3. Repeat until no vertices are left ## Calculating the In-Degrees of Vertices ```py def in_degree(g): """Calculates the in-degree of all vertices""" degree = {u:0 for u in g} for u in g: for v in g[u]: degree[v] += 1 return degree ``` ## Topological Sort Implementation ```py def toposort(g): degree = in_degree(g) ready = [u for u in degree if degree[u] == 0] output = [] while len(ready) > 0: u = ready.pop() output.append(u) for v in g[u]: degree[v] -= 1 if degree[v] == 0: ready.append(v) return output ``` # Minimum Spanning Trees ## Weighted Graphs - Recall that a *weighted graph* has numerical values, called weights, assigned to each edge - Weighted graphs are useful for modeling all sorts of real-world problems + E.g., weights could represent cost of building a power line between two points
dfa
a
a
b
b
a--b
5
c
c
a--c
7
d
d
a--d
13
c--b
11
d--c
17
## Weighted Graphs - The weights of a graph $G=(V,E)$ can be represented as a function $w:E\rightarrow\mathbb{R}_{>0}$ or as a lookup table - Thus, $w(i,j)$ is a common notation for returning the weight of the edge from vertex $i$ to vertex $j$ ## Spanning Trees - Given an undirected, connected, graph $G=(V,E)$, a **spanning tree** is a connected subgraph $T = (V,E')$ with $E'\subseteq E$ with no cycles 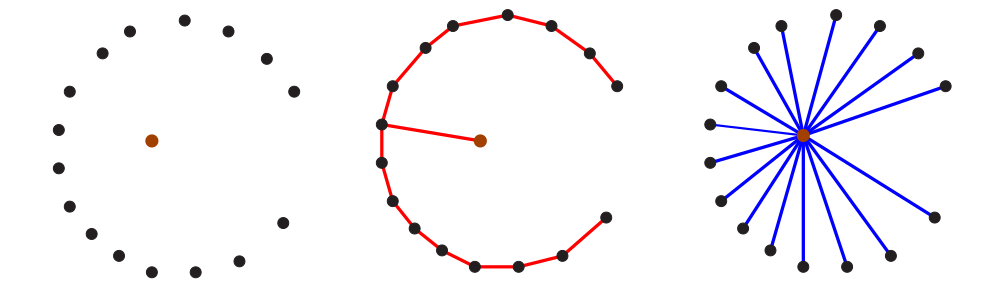 ## Minimum Spanning Trees - Finding the **minimum spanning tree** (**MST**) is extremely useful for a lot of problems 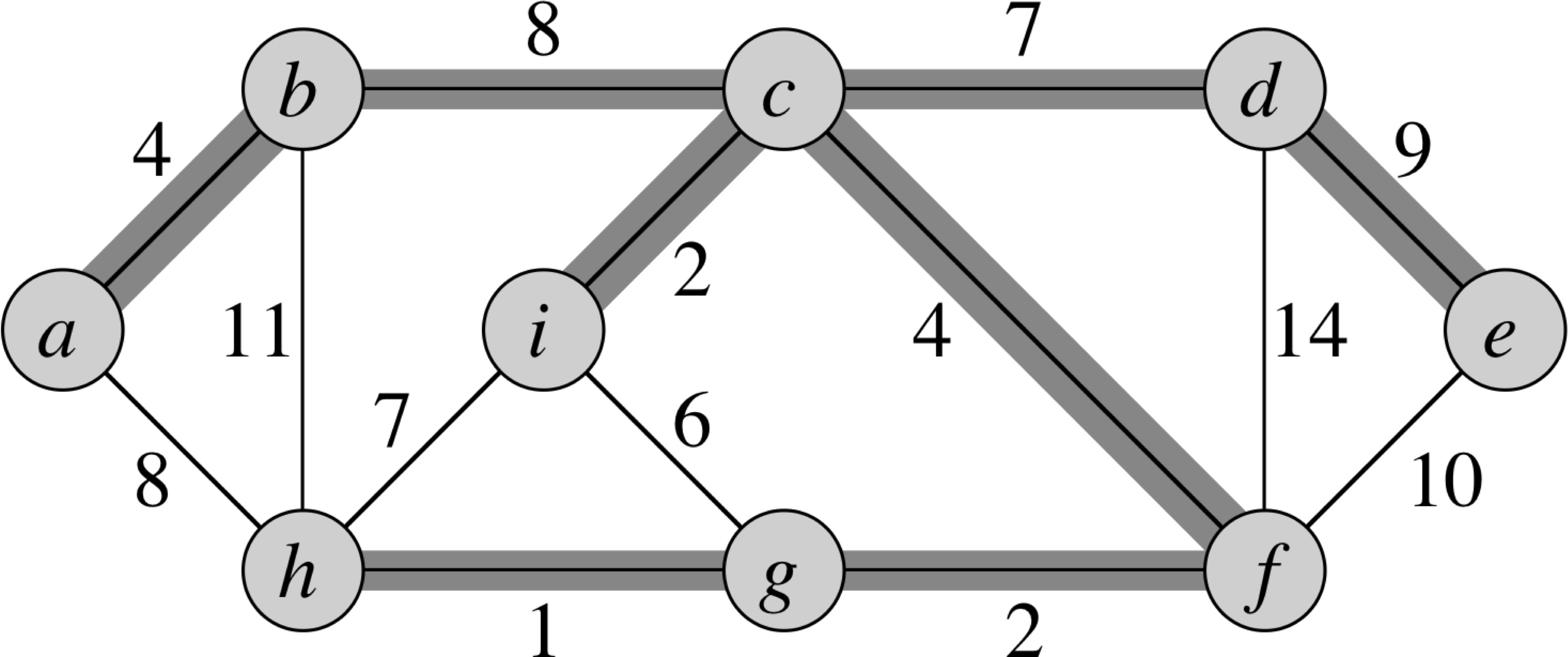 ## Formal MST Problem - **Input**: A connected, weighted, undirected graph $G = (V,E)$ with weights $w:E\rightarrow\mathbb{R}_{>0}$ - **Output**: a tree $T = (V,E')$ where $E'\subseteq E$ so that $\\sum_{e\\in E'} w(e) is minimized ## Exercise - Find a minimum spanning tree 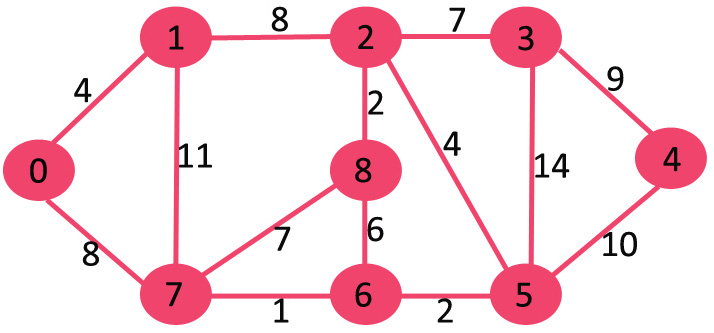 # Prim's Algorithm ## Prim's Algorithm - Prim's approach to find an MST is **greedy** - The main idea is to build up a partial MST and keep choosing the **cheapest** edge out of the tree that doesn't create a cycle ## Prim's Visualization 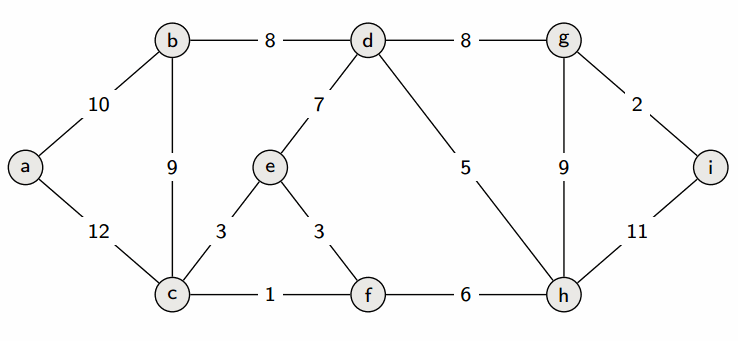 ## Prim's Pseudocode 1. Let $x\in V$ be an arbitrary vertex in $G = (V,E)$ 2. Set $S = \\{x\\}$ and $E' = \emptyset$ 3. While $S \ne V$, do the following: 1. Let $(u,v)\in E$ be the cheapest edge satisfying $u\in S$ and $v\not\in S$ 2. Add $(u,v)$ to $E'$ and $v$ to $S$ 4. Return $T = (V,E')$