# NP Completeness --- CS 137 // 2021-12-01 ## Administrivia - Exam 2 and Assignment 2 almost done grading - One short assignment to be released later today - Uplift Delivery Technology Internship Lunch-n-Learn + December 3 at Noon + Collier-Scripps Hall, Room 235 # Questions ## ...about anything? ## $P$ vs. $NP$ Video
# The Class $P$ ## The Class $P$ - Sipser discussed a class of computational problems that he called $P$ - In your own words, what is $P$? ## The Class $P$ - $P$ is the set of computational problems that are **solvable** in polynomial time - An algorithm runs in polynomial time if, in the worst case, it takes $O(n^k)$-time for some $k \ge 0$ - Intuitively, problems in $P$ are considered "easy" for a computer to solve ## Sanity Check - Suppose we have three algorithms that solve the same computational problem: + $A_1$ always takes $n$ steps + $A_2$ always takes $100n$ steps + $A_3$ always takes $n^{1000}$ steps - **Question:** All three algorithms are poly-time, does that mean in practice their performance is unnoticeable? + No! Clearly $A_1$ is the most desirable! ## Why is $P$ so important then? - The difference of $O(n)$ and $O(n^{1000})$ is substantial - The difference of $n^{1000}$ and $2^n$ is **unbelievably enormous** - Historically when an algorithm is discovered for a problem that runs in $O(n^k)$ for a large $k$, it is generally **quickly optimized** so that $k$ is small - Thus $P$ effectively captures the class of problems that can be solved practically by computers - Problems outside of $P$ are **intractable** because the resources needed to solve them are too large ## Problems in $P$ - How can we show that a problem is in $P$? + By writing an algorithm that solves it in polynomial time! - Almost every algorithm we've discussed in this class is a member of $P$ + The algorithm's we've written *prove* that those problems are in $P$ ## Problems not in $P$ - How can we show that a problem is **not** in $P$? + This is more difficult---need to show that **no algorithm exists** that can solve it efficiently - Can you think of a practical problem that you don't believe is in $P$? # The Class $NP$ ## The Class $NP$ - In your own words, what is $NP$? - $NP$ stands for **nondeterministic polynomial time** - Intuitively, $NP$ is the set of computational problems that are **verifiable** in polynomial time - What do we mean by verify? 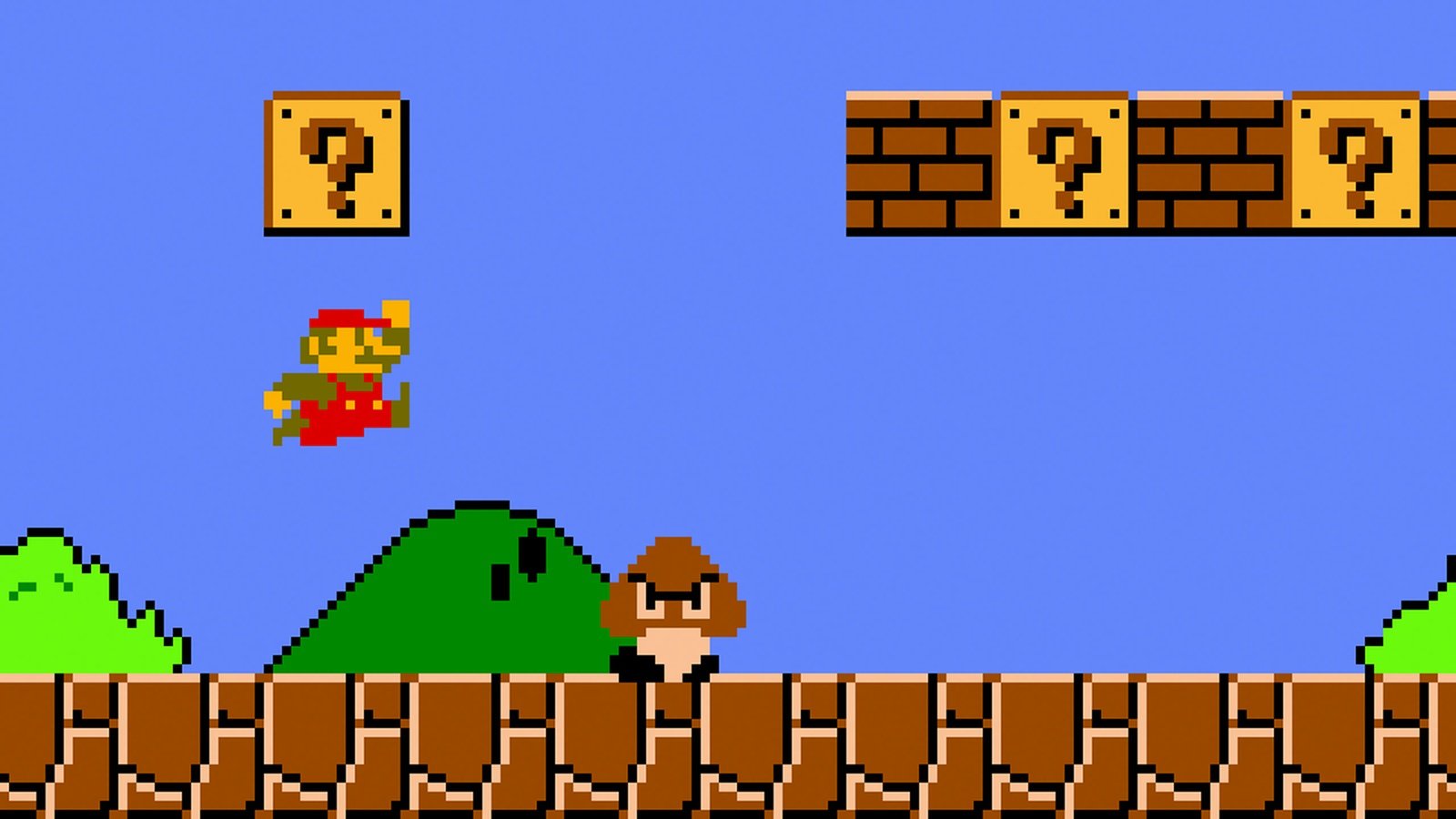 ## Super Mario Bros **Question:** How would you prove the following claim? --- - **Claim:** Super Mario Bros for NES can be completed in less than 5 minutes---from start to finish. --- - ...by doing a speedrun in under 5 minutes, recording it, and sending it to me to watch it ## Finding Versus Checking - **Finding** a route to beat Mario in five minutes is **hard**---even for a computer! - **Checking** if a speedrun is under five minutes is **easy**---at most it requires watching a five minute video ## Satisfiability - A **Boolean formula** consists of some variables, connected with AND, OR, and NOT operators: `$$\Phi = (x\lor y)\land(\lnot x\lor \lnot y)$$` - **Question:** Is there an assignment of Boolean values to `$x$` and `$y$` that causes `$\Phi$` to be true? + Yes! $x = \text{True}$ and $y = \text{False}$ - To convince a skeptic that `$\Phi$` is satisfiable, we can show them the assignment and let them **verify** it ## The Satisfiability Problem - The **Boolean satisfiability problem**, $\text{SAT}$, involves checking if a Boolean formula $\Phi$ is satisfiable - **Interesting Facts**: + We don't know if $\text{SAT}\in P$ + However, if $\Phi$ is satisfiable, there is an assignment that can be **verified** in polynomial time + Thus, $\text{SAT}\in NP$ # $P$ Versus $NP$ ## $P$ Versus $NP$ - The biggest open problem in computer science right now is the following question: Is $P = NP$? - Clearly it is true that $P \subseteq NP$ since every language *solvable* in poly time can be *checked* in poly time (just compute the answer and compare) --- - Philosophically, the $P$ versus $NP$ question is: "Is **finding** a solution harder than **verifying** a solution?" - Most computer scientists think that $P \ne NP$ ## $P$ Versus $NP$ - How can we solve the $P$ versus $NP$ problem? - It sounds so easy! 1. To show that $P \ne NP$, all we need is **one problem** that is in $NP$ but not in $P$ 2. To show that $P = NP$, all we need is a general strategy for doing Mario speedruns ## $P$ Versus $NP$ - **Theorem:** $P = NP$ if and only if general Mario levels can be speedrun efficiently - **Theorem:** $P = NP$ if and only if $\text{SAT}\in P$ - What does this mean? + It means that if you can find a fast algorithm for either of these, then you automatically get a fast algorithm **for every** $NP$ problem + In other words, both problems are **$NP$-complete** # $NP$-Completeness ## $NP$-Completeness - Problems like $\text{SAT}$ are called $NP$-complete because they are connected to the complete class of $NP$ problems - Showing that a problem is $NP$-complete sounds hard---but is actually surprisingly easy - The technique involves comparing the difficulty of two problems with a **reduction** ## Reductions - We use reductions **all the time** and don't even realize it ```py def is_even(n): """Checks whether the integer n is even""" return n % 2 == 0 ``` - Here we **reduced** one problem to another: + Problem $A$: Checking if `n` is even + Problem $B$: Checking if `n % d == r` ## Reductions - We solved an instance of $A$ by converting it to an instance of $B$, so we say that $A$ **is reducible to** $B$ - If the conversion process takes at most polynomial time, we call it a **polynomial reduction** ## Reductions - Suppose we have two problems called `Bandersnatch` and `Bo-billy` - Consider the following algorithm that solves the `Bandersnatch` problem: ```text[1-4] Bandersnatch(G) Translate G into an instance Y of Bo-billy Execute a Bo-billy algorithm on Y Return the answer from the Bo-billy algorithm ``` ## Reductions ```text[1-4] Bandersnatch(G) Translate G into an instance Y of Bo-billy Execute a Bo-billy algorithm on Y Return the answer from the Bo-billy algorithm ``` - Note that `Bandersnatch` is only correct if the translation preserves correctness - If `Bo-billy` is $O(f(n))$ and the translation is $O(g(n))$-time, then `Bandersnatch` takes $O(f(n) + g(n))$-time ## Reductions - Note that if `Bo-billy` is in $P$ and the reduction is polynomial, that immediately tells us that `Bandersnatch` is also in $P$ ## Reductions - Now suppose I have a polynomial reduction from $\text{SAT}$ to `Bo-billy`: ```text[1-4] SAT(Φ) Translate Φ to an instance Y of Bo-billy Execute a Bo-billy algorithm on Y Return the answer from the Bo-billy algorithm ``` - If someone finds a polynomial-time algorithm for `Bo-billy`, then the reduction implies that $\text{SAT}$ is also in $P$, and therefore $P = NP$! - Thus, `Bo-billy` is at least as hard as $\text{SAT}$ because $\text{SAT}$ can be solved with `Bo-billy` ## Reductions - **Main Point**: To show that a problem is hard, we can reduce an $NP$-complete problem to it - Hundreds of problems are known to be $NP$-complete, so there are a lot to pick from # Examples